Python is a versatile language that supports multiple programming paradigms, including object-oriented programming (OOP). OOP is a style of coding that helps you organize software design around data, or objects, rather than functions and logic. Understanding how to apply OOP in Python can significantly improve the readability, scalability, and maintainability of your code. Let’s dive into the basics and practical applications.
What is Object-Oriented Programming?
Object-Oriented Programming is a methodology where programs are designed by creating “objects” instances of “classes” that interact with one another. A class defines the properties (attributes) and behaviors (methods) that its objects will have.
The four fundamental principles of OOP are:
- Encapsulation: Bundling data and methods operating on that data within one unit (class).
- Abstraction: Hiding complex implementation details and showing only the necessary parts.
- Inheritance: Creating new classes from existing ones to promote code reuse.
- Polymorphism: Using a single interface to represent different data types or classes.
Python makes implementing these principles simple and intuitive.
Defining Classes and Creating Objects
A class is like a blueprint for creating objects. Here’s a simple example:
class Dog:
def __init__(self, name, breed):
self.name = name
self.breed = breed
def bark(self):
return f”{self.name} says woof!”
- “__init__” is a constructor method that initializes the object’s attributes.
- “self” represents the instance of the class.
- “name” and breed are instance variables.
- “bark” is a method that performs an action.
Creating an Object
my_dog = Dog(“Buddy”, “Golden Retriever”)
print(my_dog.bark())
Output
Buddy says woof!
Each object you create from a class can have unique attribute values.
What is Encapsulation?
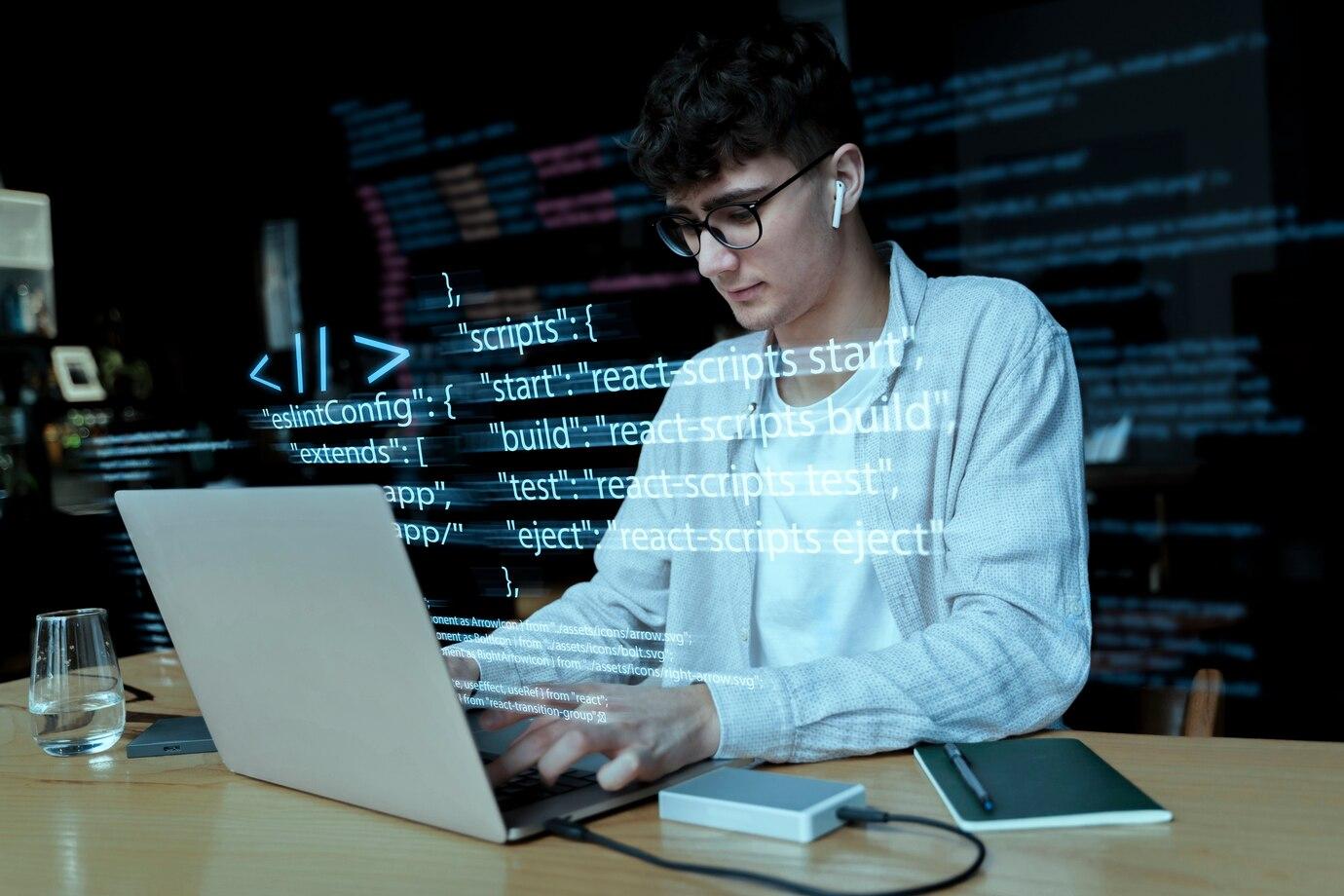
Encapsulation ensures that the internal representation of an object is hidden from the outside. You can control access to variables and methods by making attributes private using a leading underscore “_” or double underscore “__”.
Example:
class BankAccount:
def __init__(self, balance):
self.__balance = balance # Private attribute
def deposit(self, amount):
if amount > 0:
self.__balance += amount
def withdraw(self, amount):
if amount <= self.__balance:
self.__balance -= amount
else:
print(“Insufficient funds.”)
def get_balance(self):
return self.__balance
Trying to access “__balance” directly from outside the class will raise an error, protecting the internal state.
Using Inheritance for Code Reuse
Inheritance allows a class (child class) to inherit attributes and methods from another class (parent class), promoting code reuse.
Example:
class Animal:
def __init__(self, name):
self.name = name
def speak(self):
return f”{self.name} makes a sound.”
class Cat(Animal):
def speak(self):
return f”{self.name} says meow!”
class Dog(Animal):
def speak(self):
return f”{self.name} says woof!”
Creating Objects
cat = Cat(“Whiskers”)
dog = Dog(“Buddy”)
print(cat.speak()) # Output: Whiskers says meow!
print(dog.speak()) # Output: Buddy says woof!
The Cat and Dog classes override the speak method from the Animal class. This demonstrates polymorphism as well.
Polymorphism in Action
Polymorphism allows different classes to be treated as instances of the same parent class through a shared interface.
Example:
animals = [Cat(“Luna”), Dog(“Max”)]
for animal in animals:
print(animal.speak())
Each object behaves according to its own implementation of the speak method, even though the loop treats them as the parent type.
Working with Class Variables and Instance Variables
- Instance Variables: Unique to each object.
- Class Variables: Shared among all instances.
Example:
class Car:
wheels = 4 # Class variable
def __init__(self, color):
self.color = color # Instance variable
Access:
car1 = Car(“red”)
car2 = Car(“blue”)
print(car1.color) # red
print(car2.color) # blue
print(car1.wheels) # 4
print(car2.wheels) # 4
Modifying wheels at the class level affects all instances unless overridden.
Special Magic Methods
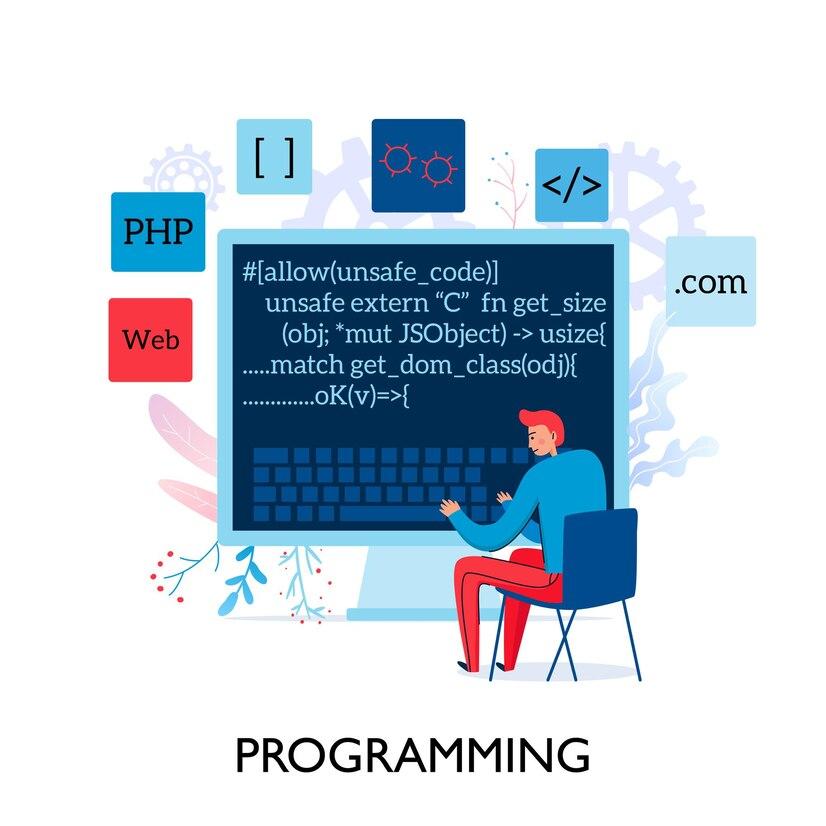
Python classes can implement special methods (also called dunder methods because they begin and end with double underscores) to customize behavior.
Common ones include:
- __str__(self): Defines the string representation of an object.
- __len__(self): Defines behavior for the built-in len() function.
- __add__(self, other): Defines behavior for the + operator.
Example:
class Book:
def __init__(self, title, pages):
self.title = title
self.pages = pages
def __str__(self):
return f”‘{self.title}’ with {self.pages} pages.”
def __len__(self):
return self.pages
Usage:
book = Book(“Python Basics”, 350)
print(book) # ‘Python Basics’ with 350 pages.
print(len(book)) # 350
Abstract Classes and Interfaces
Sometimes, you want a base class to define methods that must be implemented by subclasses. This can be achieved using the abc module (Abstract Base Classes).
Example:
from abc import ABC, abstractmethod
class Shape(ABC):
@abstractmethod
def area(self):
pass
class Circle(Shape):
def __init__(self, radius):
self.radius = radius
def area(self):
return 3.14 * self.radius ** 2
Here, any subclass of Shape must implement the area method.
Composition Over Inheritance
While inheritance is powerful, sometimes it’s better to use composition, building complex objects by combining simpler ones rather than extending classes.
Example:
class Engine:
def start(self):
return “Engine started”
class Car:
def __init__(self):
self.engine = Engine()
def start(self):
return self.engine.start()
In this example, Car uses an instance of Engine instead of inheriting from it, allowing more flexibility.
How Tambena Consulting Supports You with OOP in Python
Expert Design and Architecture
At Tambena Consulting, we specialize in building scalable, maintainable Python solutions using Object-Oriented Programming (OOP). We design clean, modular systems based on industry best practices like SOLID principles and design patterns, ensuring your software is strong and easy to extend.
Custom Python Development
Our team delivers custom OOP-based Python applications from backend systems to web platforms that are secure, reusable, and efficient. Every project is structured to meet your business needs and adapt easily as they grow.
Code Audits and Refactoring
We offer comprehensive code audits to identify weak spots and refactor procedural or outdated codebases into clean, object-oriented structures. This improves maintainability, performance, and scalability, saving you time and resources in the long run.
Agile Collaboration
Whether it’s CMS development or something else, we use agile development methods for maximum transparency and adaptability, delivering work in iterations with regular feedback loops to stay aligned with your goals.
Final Say
Object-Oriented Programming in Python provides a solid framework for designing and organizing your code. By understanding classes, objects, encapsulation, inheritance, polymorphism, and special methods, you can create strong, reusable, and elegant software systems.
When starting, practice by modeling real-world entities like cars, animals, or bank accounts into classes. As you grow comfortable, you’ll find OOP invaluable for managing larger, more complex codebases. If interested in professional services, you can contact us anytime you want. We’ll be happy to help